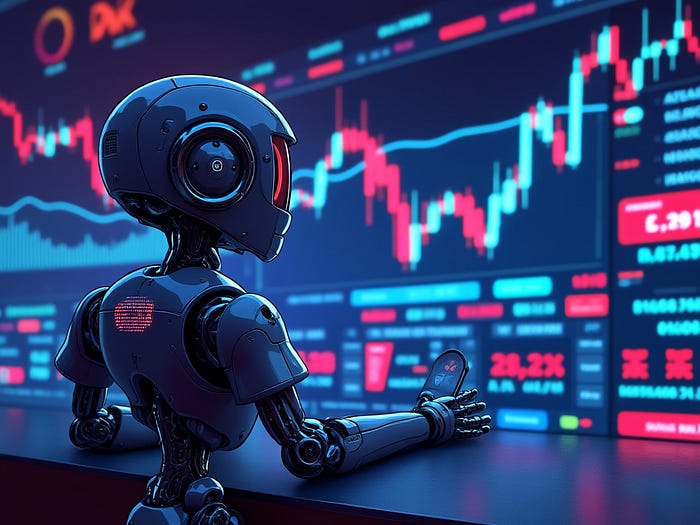
I remember the first time I heard about a trading bot.
A friend of mine casually mentioned over coffee that his investments were working for him while he slept. I chuckled, imagining little robots crunching numbers all night.
But then I thought, why shouldn’t my coffee pay for itself by the next morning?
If you’re like me — curious about the possibilities but not sure where to start — you’ve come to the right place.
The idea of a bot handling trades sounds futuristic, maybe even a bit daunting. But imagine waking up to find your investments have been hard at work overnight, potentially boosting your portfolio without you lifting a finger.
Let’s take a journey together into the world of crypto trading bots. We’ll explore how you can build your own using Python and connect it to Binance, one of the world’s leading cryptocurrency exchanges.
By the end of this little adventure, you’ll have the know-how to create a simple trading bot. Who knows? Your morning coffee might just start paying for itself.
Why Even Consider a Trading Bot?
We’ve all been there — glued to screens, watching markets that never sleep. Cryptocurrency trading can feel like a 24/7 job. But we’re only human. We need sleep, family time, and maybe a round of golf on the weekends.
A trading bot doesn’t need any of that. It can:
- Work Around the Clock: Monitor markets and execute trades anytime, day or night.
- Stick to the Plan: Follow a strategy without getting swayed by emotion or fatigue.
- Act Fast: Seize opportunities in the blink of an eye, faster than we ever could manually.
But perhaps the most compelling reason is peace of mind. Knowing that your investments are being managed, even when you’re not actively engaged, can be a game-changer.
Here’s What You’ll Need
Let’s keep things simple. You just need:
- Basic Python Knowledge: If you’ve dabbled with Python before, you’re already on your way.
- A Binance Account: It’s free to sign up, and it’s where our bot will do its work.
- API Keys from Binance: These allow your bot to interact with your Binance account securely.
- A Simple Strategy: We’ll start with something straightforward — a moving average crossover.
Step 1: Setting Up Shop
First things first, let’s get Python up and running on your computer. If you haven’t already, download Python 3.7 or later from the official website.
Next, we’ll need a few helpers — libraries that make our job easier. Open up your command prompt or terminal and type:
pip install python-binance pandas ta
This installs:
python-binance
: Communicates with Binance.pandas
: Helps us handle data neatly.ta
: Gives us tools for technical analysis.
Step 2: Making Friends with Binance
Imagine your bot trying to get into a VIP lounge — it needs the right credentials. Log into your Binance account, go to API Management, and create new API keys. Label them something like “MyTradingBot”.
You can refer to the link from Binance below to get the full steps on how to create your own API key:
https://www.binance.com/en/support/faq/how-to-create-api-keys-on-binance-360002502072
A word to the wise: Keep these keys secret. Like, James Bond secret. Don’t share them, and don’t post them anywhere public.
In your Python script, we’ll set up the connection:
from binance.client import Client
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_SECRET_KEY'
client = Client(api_key, api_secret)
Step 3: Picking a Strategy Without Overcomplicating
Let’s not reinvent the wheel. We’ll use the Moving Average Crossover strategy. Here’s the gist:
- Moving Average (MA): It’s like the average closing price over a certain number of periods.
Our Plan:
- Buy when a short-term MA crosses above a long-term MA.
- Sell when a short-term MA crosses below a long-term MA.
It’s simple, just to demonstrate how things work.
Step 4: Gathering the Goods
We need some data to work with. Let’s fetch historical prices for Bitcoin (BTC) against Tether (USDT) for the past 100 hours.
import pandas as pd
symbol = 'BTCUSDT'
interval = Client.KLINE_INTERVAL_1HOUR
limit = 100
klines = client.get_klines(symbol=symbol, interval=interval, limit=limit)
data = pd.DataFrame(klines)
# Let's clean it up and name the columns
data.columns = ['Open Time', 'Open', 'High', 'Low', 'Close', 'Volume',
'Close Time', 'Quote Asset Volume', 'Number of Trades',
'Taker Buy Base Asset Volume', 'Taker Buy Quote Asset Volume', 'Ignore']
# Convert 'Close' column to numeric
data['Close'] = pd.to_numeric(data['Close'])
Step 5: Implementing Our Strategy
Now, let’s calculate our moving averages. We take our fast MA to be 10, and our slow MA to be 30.
short_window = 10
long_window = 30
data['MA_Short'] = data['Close'].rolling(window=short_window).mean()
data['MA_Long'] = data['Close'].rolling(window=long_window).mean()
We’ll create signals based on these:
import numpy as np
data['Signal'] = 0
data['Signal'][short_window:] = np.where(
data['MA_Short'][short_window:] > data['MA_Long'][short_window:], 1, 0)
data['Position'] = data['Signal'].diff()
What we’re doing is comparing the two moving averages and setting up a signal to buy or sell.
Step 6: Time to Trade (But Carefully)
We need our bot to actually place orders. Let’s define functions for buying and selling.
def buy_order(quantity, symbol):
try:
order = client.order_market_buy(
symbol=symbol,
quantity=quantity)
print('Bought BTC')
return order
except Exception as e:
print(f'An error occurred: {e}')
return None
def sell_order(quantity, symbol):
try:
order = client.order_market_sell(
symbol=symbol,
quantity=quantity)
print('Sold BTC')
return order
except Exception as e:
print(f'An error occurred: {e}')
return None
Figuring out how much to buy:
balance = client.get_asset_balance(asset='USDT')
usdt_balance = float(balance['free'])
btc_price = float(client.get_symbol_ticker(symbol=symbol)['price'])
quantity = (usdt_balance / btc_price) * 0.99 # Let's be conservative
quantity = round(quantity, 6) # Binance allows up to 6 decimal places
Now, let’s execute trades based on our signals:
if data['Position'].iloc[-1] == 1:
buy_order(quantity, symbol)
elif data['Position'].iloc[-1] == -1:
sell_order(quantity, symbol)
else:
print('No trade signal at this time.')
Step 7: Letting It Run
We want our bot to check the market regularly. Let’s set up a loop:
import time
while True:
# Fetch fresh data
# Recalculate moving averages and signals
# Execute trades based on new signals
# Wait before checking again
time.sleep(3600) # Wait for an hour
Step 8: Test Before You Invest
This is very important. Before we let our bot handle real money, we should test it thoroughly.
Binance offers a Testnet — essentially, a playground with fake money.
Connect to the Testnet by adjusting the client (You’ll need a new testnet API):
client = Client(api_key, api_secret, testnet=True)
Run your bot in this safe environment until you’re confident everything works as expected.
A Few Words on Being Careful
Automated trading is powerful, but with great power comes great responsibility.
- Error Handling: Always handle exceptions to prevent crashes.
- Logging: Keep a log of your bot’s activities.
- Security: Store your API keys securely. Better yet, use environment variables.
- Stay Updated: Markets change. What works today might not work tomorrow.
Taking It Further
Maybe now you’re thinking, “This is great, but can I make it better?” Absolutely!
- Advanced Strategies: Explore other indicators or machine learning models.
- User Interface: Build a simple dashboard to monitor your bot.
- Risk Management: Implement stop-loss orders to protect against big losses.
- Diversification: Program your bot to trade multiple cryptocurrencies.
Reflecting on Our Journey
Building a trading bot isn’t just about the potential profits. It’s also about learning, experimenting, and maybe even having a bit of fun.
It’s like planting a garden. You prepare the soil (set up your environment), choose your plants (pick a strategy), and tend to them regularly (monitor and adjust your bot). Sometimes the weather cooperates, sometimes it doesn’t. But there’s satisfaction in the process.
Remember, investing and trading carry risks. A bot can help manage some aspects, but it’s not a magic bullet.
As you venture into this new territory, keep an open mind. Learn from both successes and setbacks. And perhaps most importantly, define what success means for you.
Additional Resources
We also offer a variety of free indicators and a premium indicator available for trial at no cost.
If you appreciate our strategy and insights, please help us grow by following our page and trying out our indicators.
To discover more about TradeDots, please glance through our comprehensive documentation with the link below: https://docs.tradedots.xyz/
🖥️ Get TradeDots Indicator: https://bit.ly/tradedots
📈 [Download] High Growth Alpha Stock List: https://bit.ly/tradedots-alphalist
📃 [Download] 2024 Forex Trading Journal: https://bit.ly/2024-trading-journal
Stay connected for more insightful blogs and updates, and join our telegram community for free trading ideas and stock watch alerts.
Twitter: https://twitter.com/tradedots
YouTube: https://youtube.com/@tradedots/
Telegram: https://t.me/tradedots_official
About TradeDots
TradeDots is a TradingView indicator that identifies market reversal patterns through the implementation of quantitative trading algorithm on price actions. Try our 7-day FREE Trial to level up your trading game.
Join us now to experience TradeDots across all trading assets!
Disclaimer: This article is for informational purposes only and does not constitute financial advice. Investing involves risks, and it’s important to conduct your own research or consult with a financial professional before making investment decisions.